Checkout options
Our front ends allow you to insert checkout options. There's a bunch of pre-configured handlers, such as (requiring) a Signature or changing who made the sale by means of SoldBy, but you can also create your own custom checkout options. You can even attach scripts to the options, to expand the functionality.
Checkout options are easily created via API, as described on this page, but you can also do so in our Checkout options chapter in Admin Suite.
A checkout option relies on the following parts:
- (optional) checkout option categories;
- checkout option handlers;
- checkout options themselves;
- (optional) custom fields;
- (optional) scripts.
To be able to manage checkout options, you need the CheckoutOptions permissions.
Check what's currently available
EVA features several pre-configured checkout option handlers, you can check which are available by means of the service GetCheckoutOptionHandlers
.
{
"Handlers": [
"Certification", // Deprecated: will be split into separate checkout options
"CustomerReferences",
"CustomFieldLine", // Although available, front-end work is still underway
"CustomField",
"Delivery", // This allows for setting (changing) a shipping method
"ElectronicInvoice", // Yields RecipientEmail and RecipientCode
"FiscalID",
"FiscalRemark",
"GiftWrapping",
"LotteryNumber",
"OrderBackendIdentifier",
"PartialShipment", // Currently unused
"PickupPoint",
"ProductSearchTemplate",
"QuickBuy",
"Remark",
"RequestedDate",
"SerialNumberValidation",
"SoldBy",
"TaxExempt",
"TaxRegistrationNumber",
"VerifyOrder", // Currently unused
"SignOrder",
"GlobalBlue"
]
}
Some checkout options will be displayed by EVA regardless when required to do so by certification providers.
A Checkout option is best combined with a Category. This is basically a folder, allowing you to sort your checkout options and prevent cluttering in your checkout page. Let's start off by checking which categories are currently available - you can run the service without specifying any values for this.
Note however that a Category is entirely optional: by not specifying it, the checkout option will become visible directly in the checkout page. A checkout option will also be visible in the checkout page directly if the checkout option matches an order requirement, in which case it will be shown right next to the tiles for the available categories.
{
"Results": [
{
"ID": 1,
"Name": "Default",
"Sequence": 0 // Defines where to show the category in your UI
}
]
}
Create a CreateCheckoutOptionCategory
In our example flow we want to create a category to which we will add a Seller option by means of the SoldBy handler.
{
"Results": [
{
"Name": "Seller",
"Description": "Everything to do with the seller",
"Sequence": 1
}
]
}
{
"ID": 2
}
In this case the Sequence property is required, since it indicates where the category should be placed in your UI. Since the Default category is currently already available as the first in the row with a value of 0, we make the Seller category the second folder by setting 1.
You can see both categories included in the example below. Note that it also contains a checkout option which does not have a CategoryID included and is therefore displayed directly.
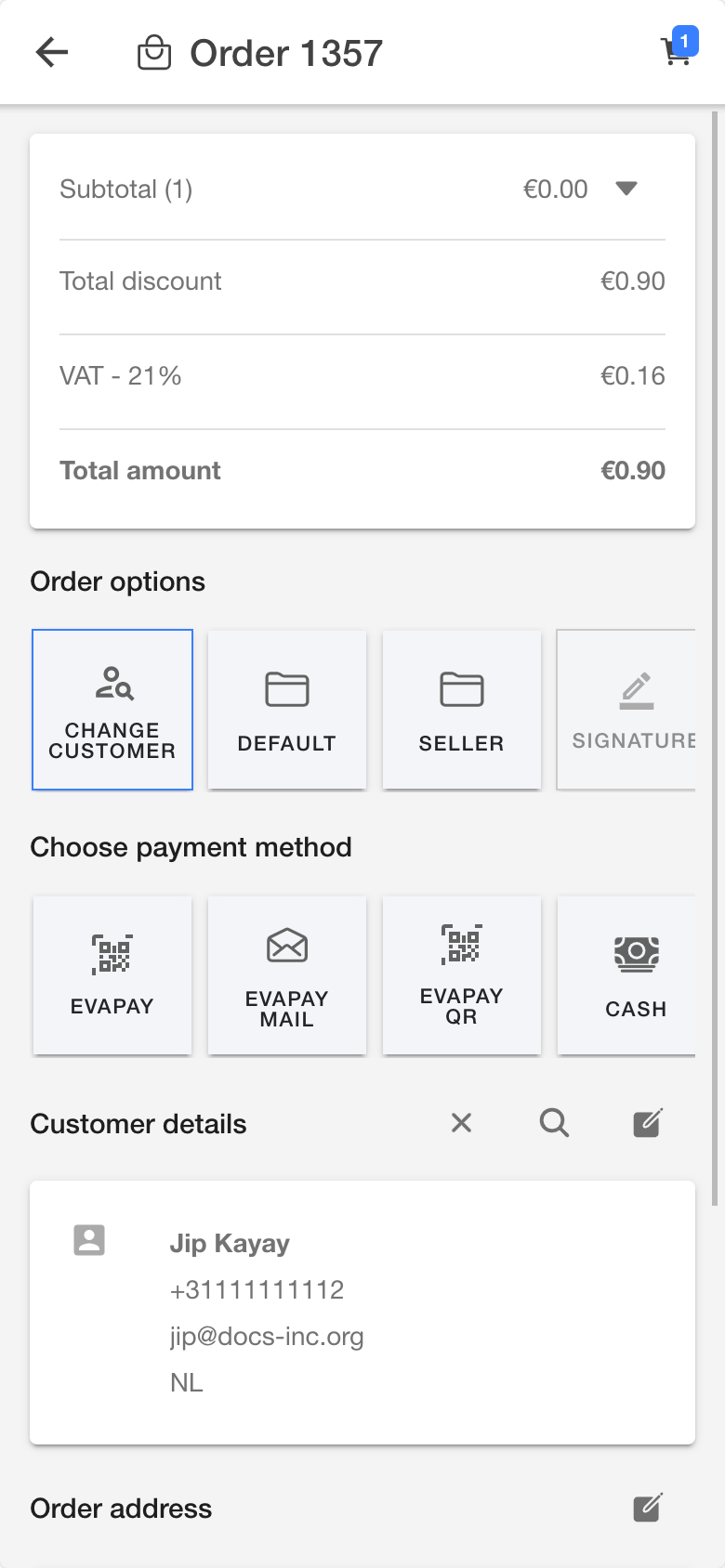
Create the CheckoutOption
We can now start creating our actual checkout options. We start off creating the mentioned Seller (SoldBy Handler) option, and additionally we will create a Signature (SignOrder Handler). We'll use the latter in the next example.
By not specifying an OU set ID, the checkout option will become available for the entire environment.
{
"CategoryID": 2, // Seller
"IsActive":"True",
"Name":"Seller",
"Description":"Assign the product sale to the employee that made it.",
"Handler":"SoldBy", // As seen in the list of handlers
"Data": null, // Can be skipped for now
"OrganizationUnitSetID": 12, // our set of physical stores
"Sequence":0 // This determines where in the category the tile will be displayed
}
{
"ID": 2
}
{
"CategoryID": 1, // Default
"IsActive":"True",
"Name":"Signature",
"Description":"Sign if needed.",
"Handler":"SignOrder", // As seen in the list of handlers
"Data": null, // Can be skipped for now
"OrganizationUnitSetID": 12, // our set of physical stores
"Sequence":2 // This determines where in the category the tile will be displayed
}
{
"ID": 3
}
Scripting
Aside from creating checkout options based on these pre-configured options, you can also create a checkout option which makes use of a custom script.
In this case we start by creating a script. We've made two easy sample scripts, the first of which will change the earlier SignOrder Handler to only apply itself for orders that are return orders.
By attaching the second one, Attached customer, the checkout option will only be shown if the order has a customer.
extend CheckoutOptions
if Order.HasReturnLines
then set output to true
end
extend CheckoutOptions
if Order.Customer has value
then set output to true
else
set output to false
end
Now we update the actual checkout option to include the ScriptID.
{
"ID": 12, //Existing Signature checkout option
"CategoryID": 1,
"IsActive":"True",
"Name":"Signature",
"Description":"Signature required for returns",
"Handler":"SignOrder",
"Data": null,
"OrganizationUnitSetID": 12,
"ScriptID": 10011, // ScriptID as seen in Admin Suite
"Sequence":1
}
The following is a screenshot taken on a return order: the Signature options has become available in the Default category.
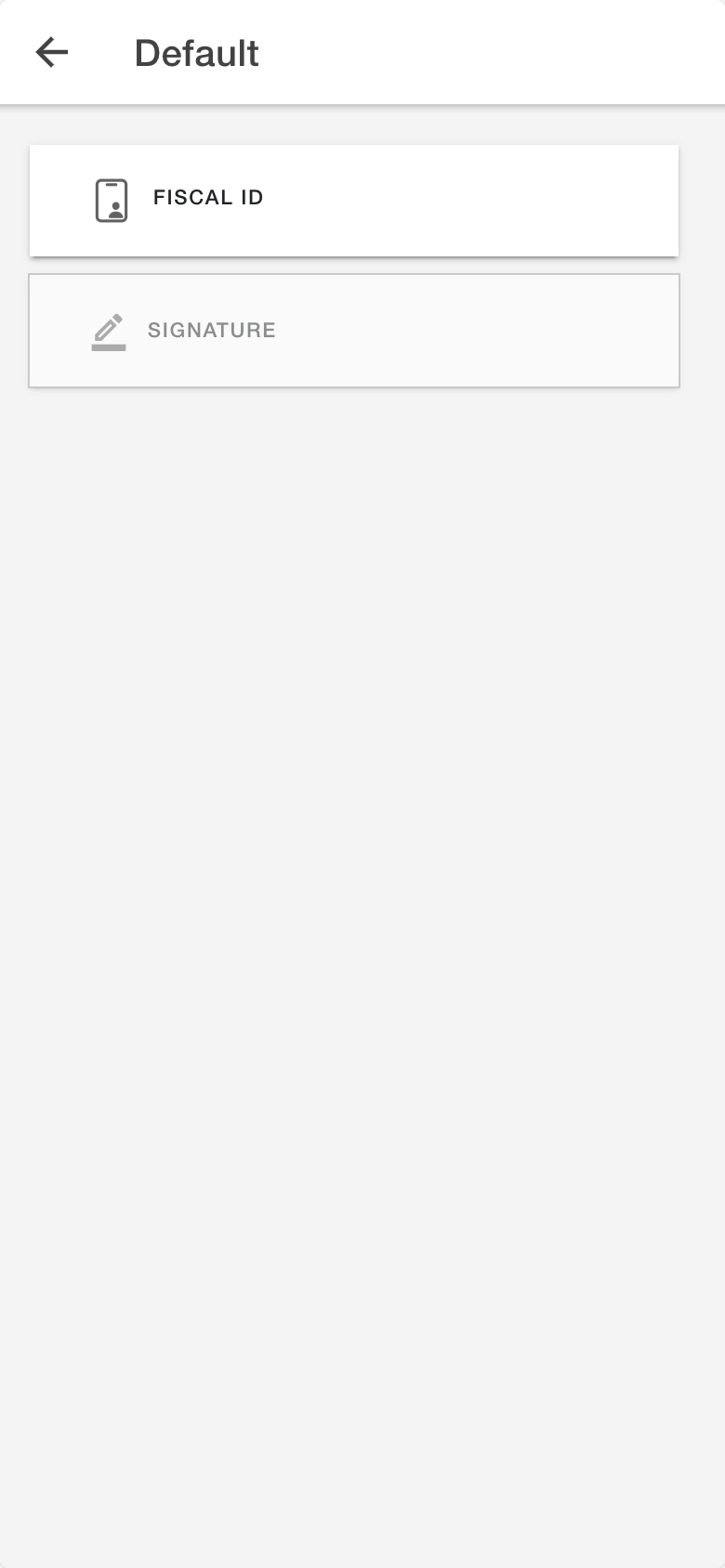
Custom fields
You can add an existing custom field - of Type Order only - to your checkout option. This requires you to use the CustomField handler. See the example below on how to add the custom field itself - pay special attention to the use of brackets in the Data object.
Although you can create a custom field which is Required, this is currently not taken into account in the front ends yet. Ergo: you cannot take advantage of a required checkout option that includes a custom field. This will however be picked up soon.
{
"CategoryID": 2, // Seller
"IsActive":"True",
"Name":"Priority",
"Description":"Make this a priority order.",
"Handler":"CustomField", // As seen in the list of handlers
"Data": {
"CustomFieldID": 6
},
"OrganizationUnitSetID": 12, // our set of physical stores
"Sequence":0 // This determines where in the category the tile will be displayed
}
{
"ID": 19
}
We've now got the Priority custom field presented as a checkout option in the Seller folder.

Results
After creating your checkout option(s), it becomes available on all front ends on your specified OU set.
You can check all your available checkout options by means of the GetCheckoutOptions
call.
{
"Options": [
{
"ID": 1,
"CategoryID": 1,
"CategoryName": "Default",
"IsActive": true,
"Name": "Delivery",
"Description": "Delivery",
"Handler": "Delivery",
"OrganizationUnitSetID": 28,
"OrganizationUnitSetName": "Checkout option: Delivery",
"Sequence": 0
},
{
"ID": 2,
"CategoryID": 1,
"CategoryName": "Default",
"IsActive": true,
"Name": "QuickBuy",
"Description": "QuickBuy",
"Handler": "QuickBuy",
"OrganizationUnitSetID": 29,
"OrganizationUnitSetName": "Checkout option: QuickBuy",
"Sequence": 0
},
{
"ID": 3,
"CategoryID": 1,
"CategoryName": "Default",
"IsActive": true,
"Name": "SignOrder",
"Description": "SignOrder",
"Handler": "SignOrder",
"OrganizationUnitSetID": 30,
"OrganizationUnitSetName": "Checkout option: SignOrder",
"Sequence": 0
},
{
"ID": 4,
"CategoryID": 1,
"CategoryName": "Default",
"IsActive": true,
"Name": "CustomerReferences",
"Description": "CustomerReferences",
"Handler": "CustomerReferences",
"OrganizationUnitSetID": 31,
"OrganizationUnitSetName": "Checkout option: CustomerReferences",
"Sequence": 0
},
{
"ID": 5,
"CategoryID": 1,
"CategoryName": "Default",
"IsActive": true,
"Name": "PartialShipment",
"Description": "PartialShipment",
"Handler": "PartialShipment",
"OrganizationUnitSetID": 32,
"OrganizationUnitSetName": "Checkout option: PartialShipment",
"Sequence": 0
},
{
"ID": 6,
"CategoryID": 1,
"CategoryName": "Default",
"IsActive": true,
"Name": "SoldBy",
"Description": "SoldBy",
"Handler": "SoldBy",
"OrganizationUnitSetID": 33,
"OrganizationUnitSetName": "Checkout option: SoldBy",
"Sequence": 0
},
{
"ID": 7,
"CategoryID": 1,
"CategoryName": "Default",
"IsActive": true,
"Name": "GiftWrapping",
"Description": "GiftWrapping",
"Handler": "GiftWrapping",
"OrganizationUnitSetID": 34,
"OrganizationUnitSetName": "Checkout option: GiftWrapping",
"Sequence": 0
},
{
"ID": 8,
"CategoryID": 1,
"CategoryName": "Default",
"IsActive": true,
"Name": "VatNumber",
"Description": "VatNumber",
"Handler": "Certification",
"OrganizationUnitSetID": 35,
"OrganizationUnitSetName": "Checkout option: Certification VatNumber",
"Sequence": 0,
"ConfigData": {
"ShowVatNumber": true
}
},
{
"ID": 9,
"CategoryID": 1,
"CategoryName": "Default",
"IsActive": true,
"Name": "FiscalID",
"Description": "FiscalID",
"Handler": "Certification",
"OrganizationUnitSetID": 36,
"OrganizationUnitSetName": "Checkout option: Certification FiscalID",
"Sequence": 0,
"ConfigData": {
"ShowFiscalID": true
}
},
{
"ID": 10,
"CategoryID": 1,
"CategoryName": "Default",
"IsActive": true,
"Name": "Italy",
"Description": "Italy",
"Handler": "Certification",
"OrganizationUnitSetID": 37,
"OrganizationUnitSetName": "Checkout option: Certification Italy",
"Sequence": 0,
"ConfigData": {
"ShowRecipientFields": true,
"ShowLotteryNumber": true
}
},
{
"ID": 11,
"IsActive": true,
"Name": "Sign here",
"Description": "Sign if needed",
"Handler": "SignOrder",
"OrganizationUnitSetID": 12,
"OrganizationUnitSetName": "Almere store",
"Sequence": 0
},
{
"ID": 12,
"CategoryID": 1,
"CategoryName": "Default",
"IsActive": true,
"Name": "Sign here",
"Description": "Sign if needed",
"Handler": "SignOrder",
"OrganizationUnitSetID": 12,
"OrganizationUnitSetName": "Almere store",
"ScriptID": 10011,
"ScriptName": "SignOrder CheckoutOption",
"Sequence": 0
},
{
"ID": 16,
"CategoryID": 2,
"CategoryName": "Seller",
"CategoryDescription": "Everything to do with employees",
"IsActive": true,
"Name": "Seller",
"Description": "Change who sold what",
"Handler": "SoldBy",
"OrganizationUnitSetID": 12,
"OrganizationUnitSetName": "Almere store",
"Sequence": 1
},
{
"ID": 17,
"CategoryID": 2,
"CategoryName": "Seller",
"CategoryDescription": "Everything to do with employees",
"IsActive": true,
"Name": "Comment",
"Description": "Add a remark",
"Handler": "Remark",
"OrganizationUnitSetID": 12,
"OrganizationUnitSetName": "Almere store",
"Sequence": 0
},
{
"ID": 19,
"CategoryID": 2,
"CategoryName": "Seller",
"CategoryDescription": "Everything to do with employees",
"IsActive": true,
"Name": "Priority",
"Description": "Make this a priority order.",
"Handler": "CustomField",
"OrganizationUnitSetID": 12,
"OrganizationUnitSetName": "Almere store",
"Sequence": 3,
"ConfigData": {
"CustomFieldID": 6
},
}
],
"Categories": [
{
"ID": 1,
"Name": "Default",
"Sequence": 0
},
{
"ID": 2,
"Name": "Seller",
"Description": "Everything to do with employees",
"Sequence": 1
}
]
}
In the following code example (either in GetShoppingCart
or GetOrder
) you can see how the checkout options and the categories they are included in are displayed in your front end.
"CheckoutOptions": [
{
"Options": [
{
"ID": 9,
"Name": "FiscalID",
"Description": "FiscalID",
"Handler": "Certification",
"ConfigData": {
"ShowFiscalID": true
},
"ValueData": {
"RecipientEmail": null,
"RecipientCode": null,
"VatNumber": null,
"FiscalID": null,
"FiscalRemark": null,
"LotteryNumber": null,
"DisplayOptions": {
"ShowRecipientFields": false,
"ShowVatNumber": false,
"ShowFiscalID": true,
"ShowFiscalRemark": false,
"ShowLotteryNumber": false
}
},
"CheckoutOptionCategory": {
"ID": 1,
"Name": "Default"
}
},
{
"ID": 12,
"Name": "Signature",
"Description": "Sign if needed",
"Handler": "SignOrder",
"ValueData": {},
"CheckoutOptionCategory": {
"ID": 1,
"Name": "Default"
}
},
{
"ID": 1,
"Name": "Default"
}, // All the checkout options listed here above are the ones contained in the Default category
{
"Options": [
{
"ID": 11,
"Name": "Signature",
"Description": "Sign here",
"Handler": "SignOrder",
"ValueData": {}
}
],
"ID": 0
}, // This one checkout options has no category
{
"Options": [
{
"ID": 16,
"Name": "Seller",
"Description": "Change who sold what",
"Handler": "SoldBy",
"ValueData": {
"OrderLineInfos": []
},
"CheckoutOptionCategory": {
"ID": 2,
"Name": "Seller",
"Description": "Everything to do with employees"
}
}
],
"ID": 2,
"Name": "Seller",
"Description": "Everything to do with employees"
},
{
"ID": 19,
"Name": "Priority",
"Description": "Make this a priority order.",
"Handler": "CustomField",
"ConfigData": {
"CustomFields": [
{
"ID": 6
}
]
},
"ValueData": {
"CustomFields": [
{
"CustomFieldID": 6,
"Name": "Priority",
"DisplayName": "Priority",
"DataType": "Bool",
"DataTypeID": 1,
"Options": {
"IsArray": false,
"IsRequired": false
},
"BackendID": "Prio1",
"IsEditableByUser": true,
"IsArray": false
}
]
},
"CheckoutOptionCategory": {
"ID": 2,
"Name": "Seller",
"Description": "Everything to do with employees"
}
}
],
"ID": 2,
"Name": "Seller",
"Description": "Everything to do with employees"
} // These are all the checkout options listed in the Sellect category
],
"AvailableCheckoutOptions": [
{
"ID": 9,
"Name": "FiscalID",
"Description": "FiscalID",
"Handler": "Certification",
"ConfigData": {
"ShowFiscalID": true
},
"ValueData": {
"RecipientEmail": null,
"RecipientCode": null,
"VatNumber": null,
"FiscalID": null,
"FiscalRemark": null,
"LotteryNumber": null,
"DisplayOptions": {
"ShowRecipientFields": false,
"ShowVatNumber": false,
"ShowFiscalID": true,
"ShowFiscalRemark": false,
"ShowLotteryNumber": false
}
},
"CheckoutOptionCategory": {
"ID": 1,
"Name": "Default"
}
},
{
"ID": 12,
"Name": "Signature",
"Description": "Sign if needed",
"Handler": "SignOrder",
"ValueData": {},
"CheckoutOptionCategory": {
"ID": 1,
"Name": "Default"
}
},
{
"ID": 16,
"Name": "Seller",
"Description": "Change who sold what",
"Handler": "SoldBy",
"ValueData": {
"OrderLineInfos": []
},
"CheckoutOptionCategory": {
"ID": 2,
"Name": "Seller",
"Description": "Everything to do with employees"
}
},
{
"ID": 19,
"Name": "Priority",
"Description": "Make this a priority order.",
"Handler": "CustomField",
"ConfigData": {
"CustomFields": [
{
"ID": 6
}
]
},
"ValueData": {
"CustomFields": [
{
"CustomFieldID": 6,
"Name": "Priority",
"DisplayName": "Priority",
"DataType": "Bool",
"DataTypeID": 1,
"Options": {
"IsArray": false,
"IsRequired": false
},
"BackendID": "Prio1",
"IsEditableByUser": true,
"IsArray": false
}
]
},
"CheckoutOptionCategory": {
"ID": 2,
"Name": "Seller",
"Description": "Everything to do with employees"
}
},
Visibility checkout options
Since checkout options are irrelevant for certain kinds of orders, we've hardcoded a script to exclude all checkout options unless the following conditions apply:
- At least 1 shippable line with quantity > 0
AND the order does not have one of the following properties:
- OrderProperties.ReturnToSupplier
- OrderProperties.Replenishment
- OrderProperties.IsTransferOrder
- OrderProperties.IsB2B
- OrderProperties.IsInterbranch
This hardcoded script will not apply anymore if you manually add a script.
Option-specific information
Although checkout options are for the most part configured by means of the services here above, there are a few options which have related settings or that benefit from additional information. Check here for a complete, alphabetical list of all options.